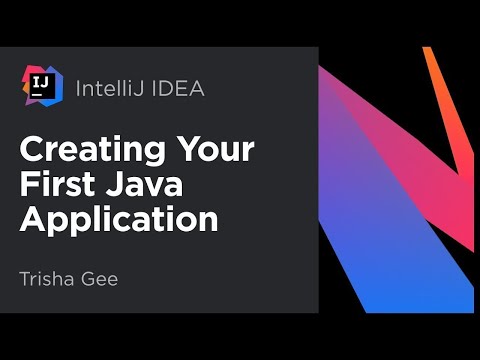
Content
- To step
- Method 1 of 3: Your first Java program
- Method 2 of 3: Hello world program
- Method 3 of 3: Input and output
- Tips
Java is an object-oriented programming language developed by James Gosling in 1991, which means that it uses concepts such as "objects" with "fields" (describing characteristics of the object) and "methods" (actions that the object can perform). Java is a "write once, run anywhere" language, which means that it is designed to run on any platform and on any Java Virtual Machine (JVM). Since Java makes extensive use of common language, it is easy for beginners to learn and understand. This tutorial is an introduction to writing programs in Java.
To step
Method 1 of 3: Your first Java program
In order to start writing programs in Java, you will first have to set up a working environment. Many programmers use integrated development environments (IDEs) such as Eclipse and Netbeans for Java programming, but you can write and compile a Java program without heavy IDEs.
Any kind of Notepad-like program is sufficient for Java programming. Hardcore programmers sometimes prefer simple text editors from the terminal, such as vim and emacs. A very good text editor that can be installed on both a Windows computer and a Linux-based machine (Ubuntu, Mac, etc.) is Sublime Text, which we will be using in this tutorial.
Make sure you have the Java Software Development Kit installed. You need this to compile your programs.
- Under Windows, if the environment variables are incorrect, you may get an error when running javac. To avoid these error messages, please refer to the Java Software Development Kit installation article for more information.
Method 2 of 3: Hello world program
We will first create a program that shows "Hello world" on the screen. Create a new file in your text editor and save it as "HelloWereld.java". HelloWorld is the name of your class, which must be the same as that of your file.
Declare your class and the main method. The main method public static void main (String [] args) is the method that is executed when the program is running. This main method has the same method declaration in every Java program.
public class HelloWorld {public static void main (String [] args) {}}
Write the line of code that will display "Hello world".
System.out.println ("Hello world.");
- Let's break this rule down into its different components:
- System tells the system that something must be done.
- out tells the system that there is an output.
- println stands for "print this line," thus telling the system that the output is a line of text.
- The quotation marks around ("Hello world.") Means that the System.out.println () method is requesting a parameter; in this case it is the string "Hello world."
- Note that there are a number of Java rules that we must adhere to here:
- Always put a semicolon at the end of a program line.
- Java is case sensitive, so you will need to put the method, variable, and class names in the correct font size or an error message will result.
- Blocks of code associated with a particular method or loop are enclosed in curly brackets.
- Let's break this rule down into its different components:
Put it all together. The final Hello World program should now look like this:
public class HelloWorld {public static void main (String [] args) {System.out.println ("Hello world."); }}
Save your file and open Command Prompt or Terminal to compile the program. Navigate to the folder where you saved HalloWereld.java and type javac HalloWereld.java. This tells the Java compiler that you want to compile HalloWereld.java. If errors have occurred, the compiler sees what you did wrong.In all other cases, the compiler will not display any messages. If you look at the directory where you saved HalloWereld.java, you should see the file HalloWereld.class. This is the file that Java uses to run your program.
Run the program. Finally we can start executing the program! In the command window or terminal, type the following: java HelloWorld. This indicates that Java should execute the class HalloWereld. You should see "Hello world" printed on the screen (in the console).
Congratulations, you wrote your first Java program!
Method 3 of 3: Input and output
We are then going to expand our Hello World program by accepting input from the user. In our Hello World program, we have printed a text string on the screen, but the interactive part of programs is the one in which the user can enter data. We are now going to expand our program with a question for the user to enter his or her name, followed by a greeting, followed by the name of the user.
Import the Scanner class. In Java there are a number of built-in libraries that we can make use of, but we will have to import them first. One of these libraries is java.util, which has a Scanner object that we need to accept input from the user. To import the Scanner class we add the following line at the beginning of our code.
import java.util.Scanner;
- This tells our program that we want to use the Scanner object in the java.util package.
- If we want to access every object in java.util, we write import java.util. *; at the beginning of our code.
Within our main method we create a new instance of the Scanner object. Java is an object-oriented language, so its concepts will use objects. The Scanner object is an example of an object with fields and methods. To be able to use the Scanner class we create a new Scanner object of which we can then fill in the fields and use its methods. You do this as follows:
Scanner userInputScanner = new Scanner (System.in);
- userInputScanner is the name of the Scanner object we just instantiated. Note that every part of the name is written in capital letters (camel case); this is the convention for naming variables in Java.
- We use the new operator to create a new instance of an object. So, in this case, we created a new instance of the Scanner object using the code new Scanner (System.in).
- The Scanner object asks for a parameter that tells the object what to scan. In this case we put the System.in as parameter. System.in tells the program to look for input from the system, which in this case is what the user types into the program.
Ask the user for input. We will have to ask the user to type something as input so that the user knows when to enter something in the console. You can do this with System.out.print or with System.out.println.
System.out.print ("What's your name?");
Ask the Scanner object to take the next line of what the user types and store it as a variable. The Scanner will always save what the user types. The following line of code will ask the Scanner to store what the user has typed as a name in a variable:
String userInputName = userInputScanner.nextLine ();
- In Java, the convention for using an object's method is the objectName.methodName (parameters) code. With userInputScanner.nextLine (), we call the Scanner object with the name we just gave it, then call its method with nextLine () without parameters.
- Note that we store the following line in another object: the String. We have named our String object userInputName.
Print a greeting on the screen to the user. Now that we have saved the user's name, we can print a greeting to the user. Do you know the System.out.println ("Hello world."); any code we wrote in the main class? All the code we've just written should be above that line. Now we can modify that line to say the following:
System.out.println ("Hello" + userInputName + "!");
- The way we use "Hello", the username and "!" linked together by "Hello" + userInputName + "!" is called String concatenation.
- What is happening here is that we are dealing with three strings: "Hello", userInputName, and "!". Strings in Java are immutable and thus cannot be changed. So when we concatenate these three strings, we essentially create a new string with the greeting.
- Then we take this new string and use it as parameter for System.out.println.
Combine it and save your work. Our code should now look like this:
import java.util.Scanner; public class HelloWorld {public static void main (String [] args) {Scanner userInputScanner = new Scanner (System.in); System.out.print ("What's your name?"); String userInputName = userInputScanner.nextLine (); System.out.println ("Hello" + userInputName + "!"); }}
Compile and run the program. Open the Command Window or the Terminal and run the same commands as for our first version of HelloWereld.java. We will have to compile the program first: javac HalloWereld.java. Then we can run it: java HelloWorld.
Tips
- Java is an object-oriented programming language, so it is useful to learn more about the fundamentals of object-oriented programming languages.
- Object Oriented Programming (OOP) has many functions specific to its paradigm. Three of these main functions are:
- Encapsulation: (encapsulation) The ability to restrict access to some parts of the object. Java has private, protected, and public modifiers for fields and methods.
- Polymorphism : the ability for objects to assume different identities. In Java, one object can become part of another object in order to use the methods of the other object.
- Inheritance: (inheritance) The ability to use fields and methods from another class in the same hierarchy as the current object.