Author:
Randy Alexander
Date Of Creation:
4 April 2021
Update Date:
1 July 2024
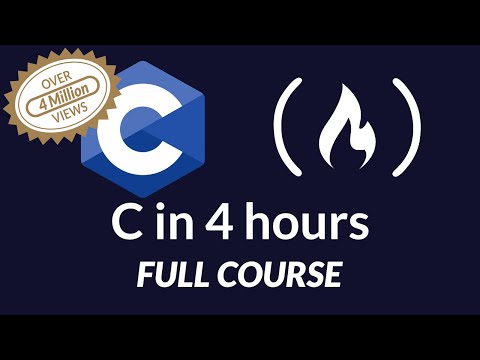
Content
C programming language is one of the oldest programming languages. This language was developed in the 70s, but it is still very strong today thanks to its low level language characteristics. Learning C is also a great way to self-learn about more complex languages; in addition, the knowledge you learn will be useful in most programming languages and can help you develop applications. To learn how to start programming in C, see Step 1 below.
Steps
Method 1 of 6: Get ready
Download and install the compiler. C code needs to be compiled by a decoder in order to decode the codes into signals that the machine can understand. Compilers are usually free, and there are many different compilers for different operating systems.- For Windows, try Microsoft Visual Studio Express or MinGW.
- For Mac, XCode is one of the best C compilers.
- For Linux, one of the most popular options is gcc.
Learn the basics. C is one of the old programming languages and can be very powerful. The language was designed for Unix operating systems, but was later ported and extended for most operating systems. And the modern version of C is C ++.- C is mainly composed of functions, and in these functions you can use variables, conditional statements, and loops to store and manipulate data.
Check out some basic code. Take a look at the (very) basic program below to get a better understanding of how the different parts of the language work together and also understand how the programs work.- Comeinand #include is executed before the program starts and loads the libraries that contain the functions you need. In this example, stdio.h allows us to use functions printf () and jaw getchar ().
- Comeinand {int main () tells the compiler that the program is running a function called "main" and it will return an integer when it finishes. All C programs run a "main" function.
- {} indicates that everything inside of them is part of the function. In this case, they denote that everything inside is part of the "main" function.
- Jaw printf () displays the text in parentheses on the user's screen. The quotes ensure that the inner string is printed literally. Chain n tells the compiler to move the cursor to the next line.
- ; denotes the end of a line. Most lines of C code must end with a semicolon.
- Comeinand getchar () requires the compiler to wait for keyboard input before moving on. This is useful because many compilers will run the program and close the window immediately. As such, this command will keep the program from being closed until a key is pressed.
- Comeinand return 0 (return) denotes the end of the function. Note how the "main" function is a function int. This means that it will need an integer returned when the program ends. The number "0" indicates that the program was correctly executed; If any other number is returned, it means the program has encountered an error.
Try compiling the program. Enter the code into the code compiler and save it as a " *. C" file. Compile this code in your compiler, usually by clicking the Build button or the Run button.
Always comment on your code. Notes are part of the code and will not be compiled, but these notes help you explain what is happening. This point is useful when you want to remind you what your code is for, and it also helps other developers looking at your code better.- To make notes in C, put /* at the beginning of the notes section and end with */.
- You can take notes about everything, not just the most basic of your code.
- You can use the notes section to quickly remove sections of code without deleting. Simply enclose the code you want to delete with flash tags and then compile. If you want to add the code back, remove these tags.
Method 2 of 6: Using variables
Understand the functions of variables. Variables allow you to store data, even computations in the program, or data from user input. Variables must be defined before you can use them, and there are many different types of variables to choose from.- Some of the more popular include int, char, and float. Each variable will store a different data type.
Learn how variables are declared. Variables must be set, or "declared", before being used by the program. You declare a variable by entering a data type followed by the variable's name. For example, here are all valid variable declarations:- Note that you can declare multiple variables on the same line, as long as they are of the same type. You just need to separate the names of the variables together with commas.
- Like many other lines in C, each variable declaration line needs to end with a semicolon.
Find the location of the variable declaration. Variables must be declared at the beginning of each code block (The code sections are in brackets {}). If you try to declare a variable at the end of the block, the program won't work correctly.
Use variables to store user data. Now that you have some basic knowledge of how variables work, you can write a simple program to store user input data. You will use another function in the program, called scanf. This function looks for input provided with a specific value.- Chain "% d" request scanf find integers in the user input.
- Comeinand & before the variable x for scanf know where to find the variables to replace it, and store the integers in the variable.
- Final order printf re-read the input integer to the user.
Manipulating variables. You can use mathematical expressions to manipulate data that you have stored in your variables. The most important difference to remember with mathematical expressions is a sign = means set the value of the variable, while 2 signs == ie comparing values on two sides to see if they are equal. advertisement
Method 3 of 6: Use conditional statements
Learn the basics of conditional statements. The conditional statement is the control element for most programs. These are statements identified as TRUE or FALSE, and then executed based on the result. The most basic statement is the command if.- TRUE and FALSE in C will behave differently than what you may have used. The TRUE statement always ends with a nonzero number. When you perform the comparison, if the result is TRUE, "1" will be returned. If the result is FALSE, "0" is returned. Knowing this point will help you understand how IF statements are processed.
Learn the basic conditional operators. Conditional statements revolve around the use of mathematical operators to compare values. Below is a list of the most commonly used conditional operators.
Write the basic IF statement. You can use the IF statement to determine what the program should do next after the statement has been evaluated. You can combine the if statement with the following conditional statements to make better choices, but for now write a simple statement to get used to them.
Use ELSE / ELSE IF statements to extend your criteria. You can build on an IF statement using an ELSE statement and an ELSE IF statement to handle different results. The ELSE statement runs if the IF statement is FALSE. ELSE IF statements allow you to put multiple IF statements into one block of code to handle different scenarios. See the example program below for a better understanding of how they interact.- The program takes data from the user and passes it through IF statements. If the metric meets the first statement, then the statement printf first be returned.If it fails to respond to the first statement, it is passed through the ELSE IF statements until it finds the correct one. If it doesn't match any of the statements, it passes the ELSE statement at the end.
Method 4 of 6: Learn Loops
Understand how loops work. Loops are one of the most important aspects of programming because they allow you to repeat blocks of code until specific conditions are met. This can make repetitive operations very easy to do and prevent you from having to rewrite new conditional statements every time you want to do something.- There are three main types of loops: FOR, WHILE, and DO ... WHILE.
Use a FOR loop. This is the most common and useful type of loop. The loop will continue to run the functions until the conditions set in the FOR loop are met. The FOR loop requires three conditions: variable initialization, conditional expressions to be met, and how variables can be updated. If you don't need all of these conditions, you still need to leave a blank space with a semicolon, otherwise the loop will run forever.- In the above program, y is set to 0, and the loop continues to run for as long as the value is on y less than 15. Each value y is printed, then value y will be added 1 and the loop will be repeated. Until y = 15, the loop will be destroyed.
Use a WHILE loop. The WHILE loop is simpler than the FOR loop. This type of loop has only one conditional expression, and the loop will work as long as the conditional expression is true. You don't need to initialize or update the variable, although you can do it in the main part of the loop.- Comeinand y ++ will add 1 to the variable y each time the loop is executed. When turning y reaches 16 (remember, this loop will keep running as long as that value y less or equal 15), the loop is discontinued.
Use loop DO...WHILE This loop is useful for loops that you want to make sure to run at least once. In the FOR and WHILE loops, the conditional expression is checked at the beginning of the loop, i.e. it cannot pass and fails immediately. Since the DO ... WHILE loop checks for the condition at the end of the loop, it will ensure that the loop executes at least once.- This loop will display the message even though the condition is FALSE. Out y is set to 5 and the WHILE loop is set to run when y is not equal to 5, so the round ends. The message is printed from the time the condition is not checked until the end.
- The WHILE loop in the DO ... WHILE setting must be terminated with a semicolon. This is the only time a loop ends with a semicolon.
Method 5 of 6: Using functions
Learn the basics of functions. Functions are independent blocks of code that can be called by other parts of the program. These functions make the program easy to repeat code, and help make the program simple to read and change. Functions can include all of the techniques previously learned in this article, and even others.- Current main () At the beginning of all of the above examples is a function, eg getchar ()
- The functions are essential to make the code efficient and easy to read. Make good use of functions to organize your program.
Start with sketching. Functions are best created when you outline what you want it to accomplish before you start actually coding. The basic syntax for functions is "return_type name (argument1, argument2, etc.)"; For example, to create a function that adds two numbers:- This will create a function that adds two integers (x and y) together and then returns the sum that is also an integer.
Add the function to the program. You can use sketch to create a program that takes the two integers the user entered and then adds them together. The program determines how the "add" function works and uses it to manipulate the inputs.- Note that the outline is still at the beginning of the program. This tells the compiler what you expect when the function is called and what the result is. This is only necessary if you want to define end-of-program functions. You can set the function add () (plus) before the function main () and the result will be the same without an outline.
- The actual function of the function is defined at the end of the program. Jaw main () Collect the integers from the user and then send them to the function add () to process. Jaw add () performs the add function and then returns the results given main ()
- At this moment add () has been defined, can be called anywhere in the program.
Method 6 of 6: Continue to dig deeper
Find a few books about C programming. This article covers the basics, but just the surface of C programming and all related knowledge. A good reference book will help you to solve many problems and save you from headaches with difficult problems later.
Join some communities. There are many communities, both online and in the real world, for programming and all programming languages. Find a number of C programmers with similar passions to exchange codes and ideas with, and you will find yourself learning a lot soon.- Attend some hack-a-thons competitions if possible. These are events where groups and individuals come up with programs and solutions, and often drive creativity within certain timelines. You can meet a lot of good programmers this way, and hack-a-thon competitions are held around the globe.
Take some classes. You don't have to go back to school to get a Computer Science degree, but you can take a few classes where you can learn more. There is nothing better than getting practical help from people fluent in programming languages. Usually, you can find classes at your local community centers and junior high schools, and some universities allow you to take computer science programs without having to register. .
Consider learning C ++. Once you have a good understanding of the C programming language, you can start learning C ++. This is a more modern version of C, and allows for a lot more flexibility. C ++ is designed with object processing in mind, and can allow you to create more powerful programs for most operating systems. advertisement
Advice
- Always add notes to your program. Not only does this section help others see its source code, but it also helps you remember what you are writing and why you wrote it. At the moment of coding, you probably know what you are writing it for, but after two or three months, you probably won't remember much about the purpose and reason for knowing the code.
- Always remember to end a statement like printf (), scanf (), getch (), etc. with a semicolon (;) but never insert it after a control statement like 'if', 'while' loop, or 'for'.
- When you get a syntax error while compiling, if you are having trouble, look for the error you are seeing on Google (or other search engine). Chances are someone has had the same problem as you and posted a solution.
- Your source code needs the * .c extension for the compiler to understand that it is a C source file.
- Has iron grinding makes perfect. The more you practice writing programs, the better you will become. So starting with simple and short programs until you become more proficient and confident can move on to a more complex type of program.
- Try to learn to build logic. It helps to solve various problems while coding.