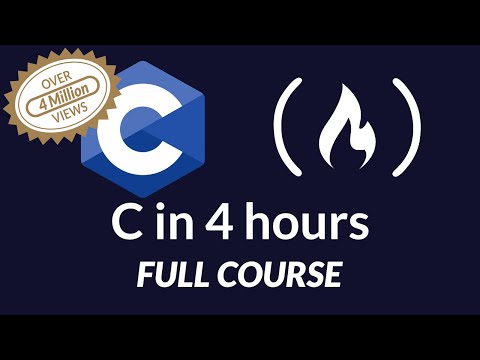
Content
- To step
- Part 1 of 6: The preparations
- Part 2 of 6: Using variables
- Part 3 of 6: Conditional statements
- Part 4 of 6: Loops
- Part 5 of 6: Using features
- Part 6 of 6: Keep learning
- Tips
C is one of the older programming languages. It was developed in the 1970s but is still known as a powerful language as it is a low-level language close to machine language. Learning C is a great introduction to programming in more complex languages, and the knowledge you gain is useful for almost any programming language, and can help you eventually get into app development. Read on to get started programming in C.
To step
Part 1 of 6: The preparations
Download and install a compiler. C code must first be compiled by a program that can interpret the code and convert it into a language that the machine can understand. Compilers are usually available for free, and you can download different compilers for each operating system.
- For Windows, Microsoft Visual Studio Express or MinGW.
- For Mac, XCode is one of the best C compilers.
- On Linux, gcc is one of the most popular options.
The basics. C is one of the older programming languages, but very powerful. It was initially designed for Unix operating system, but has eventually become common on almost every system. The "modern" version of C is C ++.
- C consists of all functions, and within these functions you can use variables, conditional statements and loops for storing and manipulating data.
Check out a few lines of simple code. Go through the (very) simple program below to get a first idea of how the different parts of the language work together, and how programs function.
#include stdio.h> int main () {printf ("Hello, World! n"); getchar (); return 0; }
- The assignment #include is placed at the beginning of a program, and loads libraries (code libraries) containing the functions you need. In this example stdio.h sure you printf () and getchar () can use.
- The assignment int main () tells the compiler that the program uses the "main" function and that it will return an integer after executing it. All C programs run as a "main" function.
- The signs {} indicate that everything inside is part of the "main" function.
- The function printf () shows the contents of the brackets on the user's screen. The quotation marks ensure that the string is printed literally. The n tells the compiler to move the cursor to the next line.
- The sign ; indicates the end of a line. Most lines of code should end with a semicolon.
- The assignment getchar ()tells the compiler to wait for a keystroke before continuing. This is useful because many compilers run the program and then immediately close the window. This prevents the program from exiting until a key is pressed.
- The assignment return 0 indicates the end of the function. Note that the "main" function is a int function is. This means it should return an integer once the program is done. A "0" indicates that the program has been executed correctly; any other number indicates an error has been detected.
Try to compile the program. Enter the code in your code editor and save it as a " *. C" file. Now compile this with your compiler, usually by pressing Build or Run.
Always include an explanation with your code. This should be a regular part of the program, but it will not be compiled. This tutorial will help you remember what the code is for, and serve as a guide for programmers looking at and / or wanting to use your code.
- To add a comment in C, place a /* at the beginning of the comment and a */ at the end.
- Comment anywhere except the most standard parts of your code.
- Comments can be used to quickly hide parts of the code without removing them. Surround the code by putting it in comment tags and then compile the program. If you want to use the code again, remove the tags.
Part 2 of 6: Using variables
The function of variables. Variables allow you to store data, either calculation results or user input. Variables must be defined before you can use them, and there are several types to choose from.
- Some of the more common variables are int, char and float. Each of these stores a different data type.
Learn how variables are declared. Variables must first be given a certain type, or "declared", before they can be used in a C program. You declare a variable by specifying the data type, followed by the name of the variable. For example, the following declarations are all valid in C:
float x; char name; int a, b, c, d;
- Note that you can declare multiple variables on the same line as long as they are of the same type. The only thing is that you separate the variables with a comma.
- Like many lines in C, it is mandatory to separate each variable declaration with a semicolon.
Know where to declare the variables. Variables must be declared at the beginning of a code block (The parts of code that are enclosed in {}). If you try to declare a variable later, the program will not function properly.
Make use of variables to store user input. Now that you know the basics of how variables work, you can write a simple program that accepts and stores input from the user. You use another function of C for this, namely scanf. This function searches for special values in a string.
#include stdio.h> int main () {int x; printf ("Please enter a number:"); scanf ("% d", & x); printf ("The number is% d", x); getchar (); return 0; }
- The "% d" string / string scanf to search for an integer in user input.
- The & for the variable X tells scanf where to find the variable to change it, and store the integer as that variable.
- The last command printf reads the variable and displays the result to the user.
Editing the variables. You can edit the data you have stored in the variables using mathematical expressions. The main distinction to remember for the mathematical expressions is that a single one = stores the value of the variable, while == the values on both sides of the character to make sure they are the same.
x = 3 * 4; / * assign "x" to 3 * 4, or 12 * / x = x + 3; / * this adds 3 to the previous value of "x", and sets the new value as variable * / x == 15; / * checks if "x" equals 15 * / x 10; / * checks if the value of "x" is less than 10 * /
Part 3 of 6: Conditional statements
Understand the basics of conditional statements. Conditional statements are what lies at the heart of most programs. These are statements that are either TRUE or FALSE, and return a result accordingly. The simplest of these statements is it if statement.
- TRUE and FALSE work differently in C than what you might be used to. TRUE statements always end with matching a nonzero number. When you perform comparisons and the result is TRUE, a "1" is returned. If the result is FALSE, a "0" is returned. Understanding this helps to work with IF statements.
Learn the standard conditional operators. Conditional statements revolve around the use of mathematical operators that compare values. The following list contains the most commonly used conditional operators.
> / * greater than * / / * less than * /> = / * greater than or equal to * / = / * less than or equal to * / == / * equal to * /! = / * not equal to * /
10> 5 TRUE 6 15 TRUE 8> = 8 TRUE 4 = 8 TRUE 3 == 3 TRUE 4! = 5 TRUE
The basic IF statement. You can use IF statements to determine what the program should do after the statement has been evaluated. You can combine this with other conditional statements to create powerful, complex functions, but we'll keep it easy to get used to for now.
#include stdio.h> int main () {if (3 5) printf ("3 is less than 5"); getchar (); }
Use ELSE / ELSE IF statements to extend your conditions. You can build on the IF statements by using ELSE and ELSE IF statements to process different results. ELSE statements are only executed if the IF statement is FALSE. ELSE IF statements allow you to use multiple IF statements within the same code block and thus create more complex conditions. See the sample program below to learn how this works.
#include stdio.h> int main () {int age; printf ("Enter your age:"); scanf ("% d", & age); if (age = 12) {printf ("You are still a child! n"); } else if (age 20) {printf ("It's great being a teenager! n"); } else if (age 40) {printf ("You are still young at heart! n"); } else {printf ("With age comes wisdom. n"); } return 0; }
- The program takes the input from the user and runs it through a number of IF statements. If the number satisfies the first statement, it becomes the first printf statement is returned. If it does not satisfy the first statement, it checks whether one of the following ELSE IF statements satisfies until you find something that works. If none of the statements are satisfactory, the last ELSE statement is executed.
Part 4 of 6: Loops
How loops work. Loops are one of the most important aspects of programming, as they allow you to repeat blocks of code until certain conditions are met. This makes implementing repetitive actions very easy, and there is no need to write new conditional statements every time you want something to happen.
- There are three different loops: FOR, WHILE and DO ... WHILE.
The FOR loop. This is the most common and useful loop type. This will keep a function running until certain conditions are met, as specified in the FOR loop. FOR loops require 3 conditions: initializing the variable, the condition to be met, and the variable to be updated. If you don't need all these conditions, you will have to put an empty space with a semicolon, otherwise the loop will go on indefinitely.
#include stdio.h> int main () {int y; for (y = 0; y 15; y ++;) {printf ("% d n", y); } getchar (); }
- In the above program y set to 0, and the loop will continue as long as the value of y is less than 15. Any time the value of y is printed on the screen, 1 is added to the value of y and the loop is repeated. Does that count y = 15, the loop will be interrupted.
The WHILE loop. WHILE loops are a bit simpler than FOR loops. These have only 1 condition and the loop continues as long as that condition is met. There is no need to initialize or update a variable, but you can do that in the loop itself.
#include stdio.h> int main () {int y; while (y = 15) {printf ("% d n", y); y ++; } getchar (); }
- The y ++ command adds 1 to the variable yevery time the loop is executed. If y arrived at 16 (remember that this loop continues as long as y "less than or equal to" 15), the loop will be stopped.
The DO...WHILE loop. This loop is very useful for loops of which you want to make sure that they are completed at least once. In FOR and WHILE loops, the condition is checked at the beginning of the loop, which means that the loop is completed or not. DO ... WHILE loops only check if the condition is fulfilled at the end and are therefore executed at least once.
#include stdio.h> int main () {int y; y = 5; do {printf ("The loop is running! n"); } while (y! = 5); getchar (); }
- This loop will display the message even if the condition is FALSE. The variable y is set to 5 and the WHILE loop will continue for as long y not equal to 5, after which the loop ends. The message was already displayed on the screen because it is only checked at the end that the condition is met.
- The WHILE loop in DO ... WHILE must end with a semicolon. This is the only time a loop ends with a semicolon.
Part 5 of 6: Using features
The basic knowledge of functions. Functions are self-contained blocks of code that can be called from another part of a program. This makes it a lot easier to repeat code and programs easier, both to read and to modify. Functions use all the techniques described above, and even other functions.
- The rule main () at the beginning of all previous examples is a function, as well getchar ()
- Functions are intended to make reading and writing code more efficient. Make good use of features to streamline your program.
Start with a brief description. Functions are best designed by first describing what you want to achieve before you start with the actual coding. The basic syntax of a function in C is "return_type name (argument1, argument2, etc.);". For example, to create a function that adds two numbers, do the following:
int add (int x, int y);
- This creates a function for adding two integers (X and y), and the sum returns as an integer.
Add the function to a program. You can use the short description to create a program for adding two user-entered integers. The program will define how the "add" function works and use it to process the entered numbers.
#include stdio.h> int add (int x, int y); int main () {int x; int y; printf ("Please enter two numbers to add:"); scanf ("% d", & x); scanf ("% d", & y); printf ("The sum of the numbers is% d n", add (x, y)); getchar (); } int add (int x, int y) {return x + y; }
- Note that the short description is at the beginning of the program. This tells the compiler what to expect when the function is called and what it will return. This is only necessary if you want to define the function later in the program. You can also add () define for the function main () so the result is the same as without the short description.
- The operation of the function is defined at the end of the program. The function main () obtains the user's integers and then forwards them to the function add () to be processed. The function add () then returns the result to main ()
- Now add () is defined, it can be called anywhere within the program.
Part 6 of 6: Keep learning
Go through some books on programming in C. This article will only go into the basics, and that's just the tip of the iceberg called C and everything that goes with it. A good book will help solve problems and can save you a lot of the headaches later on.
Join a group. There are many groups, both online and in the real world, dedicated to programming and programming languages of all kinds. Find a few like-minded C programmers to exchange code and ideas with, and you will find that in a short amount of time you have learned a lot more than you thought possible.
- Go to some hack-a-thons if possible. These are events where teams and individuals have to come up with the solution and the corresponding program for a problem within a certain time, something that requires a lot of creativity. You can meet many great programmers, and hack-a-thons are organized all over the world.
Take a course. You really don't have to go back to school to train as a programmer, but it doesn't hurt to take a course and really ramp up your learning pace. Nothing can compete with direct help from people who are very well versed in a particular subject. You can often find a course nearby, or try searching for an online course.
Also consider learning C ++. Once you've mastered C, it doesn't hurt to move on to C ++. This is the more modern variant of C, and offers much more flexibility. C ++ is designed for working with objects, and being able to work with C ++ allows you to write powerful programs for almost any operating system.
Tips
- Always comment on your programs. Not only does this help others understand your source code, but it is also to help you remember what you encoded and why. You may now know what you are doing, but after about 2-3 months, chances are that you have no idea anymore.
- Don't forget to end a statement like printf (), scanf (), getch (), etc with a semicolon (;) but never put it behind statements like "if", "while" or "for" loops.
- If you encounter a syntax error during compile time and you get stuck, use your favorite search engine to find out what the error message means. Chances are that someone else has already posted a solution for the same problem.
- The source code must have a *. C extension so that the compiler knows it is a C file.
- Remember, practice makes perfect. The more you practice writing programs, the better you will become. So start with simple, short programs until you have a solid foothold, then move on to the more complex programs.
- Learn about logic. This helps solve various problems while you are coding.