Author:
Tamara Smith
Date Of Creation:
23 January 2021
Update Date:
1 July 2024
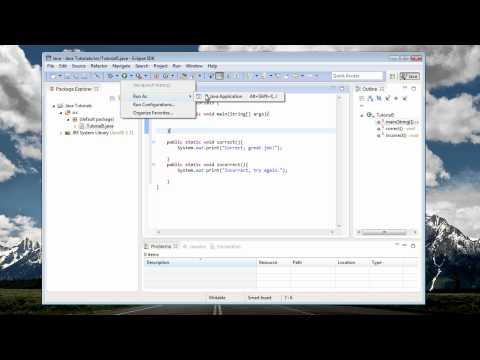
Content
When you start programming in Java, there are many new concepts to learn. There are classes, methods, exceptions, constructors, variables, etc., and it can be overwhelming at times. So it is best to learn the language step by step. In this article you will learn how to call a method in Java.
To step
A method is the equivalent of a function in languages such as C, which makes code reuse easier. A number of statements together make a method, and this method can be called by another statement. When a method is called, all statements that are part of that method will be executed. For example, consider this method: "public static void methodExample () {}". It does not contain any code yet, but there are three keywords for the method name. These are public, static and void.
The word public before the method name means that the method itself can be called from anywhere, such as classes or even from other packages (files), as long as you import the class (class). There are three other words that can replace public. These are protected and private. If a method is protected, then only this class and subclasses (classes that use this as a basis for further code) can call the method. If a method is private, then the method can only be called from within the class itself. The last keyword is basically not even a word. Use this word if you don't have anything else instead of public, protected, or private. This is called the "default", or package-private. This means that only the classes in the same package can call the method.
The second keyword, static, means that the method belongs to the class and is not an instance of the class (object). Static methods must be called using the class name: "ExampleClass.methodExample ()". However, if there is no static, then the method can only be called by an object. For example, with a class named ExampleObject and a constructor (for creating objects), we can create a new object with the code ExampleObject obj = new ExampleObject (); and then call the method with "obj.methodExample () ; ".
The last word before the method name is void. The word void means that the method returns nothing (when you run the method). If you want a method to return something, replace the word void with a datatype (primitive or reference type) of the object (or primitive type) you want to return. Then add the return code and an object of that type somewhere at the end of the method's code.
When calling a method that does return something, you can use whatever is returned. For example, if someMethod () returns an integer, you can give an integer the value of what was returned with the code "int a = someMethod ();"
Some methods require a parameter. A method that requires a parameter or an integer looks something like this: someMethod (int a). When using such a method, you write the method name, and then an integer in parentheses: someMethod (5) or someMethod (n) if n is an integer.
Methods can also have multiple parameters separated by commas. If the someMethod method requires two parameters, int a and Object obj, then write this as "someMethod (int a, Object obj)". To use this new method, it would be called by the method name, followed by an integer and an Object in parentheses: someMethod (4, thing) where thing is an Object.
Tips
- When you call a method that returns something, you can call another method based on what that method returns. Let's say we have a getObject () method, which returns an object. In the Object class, there is a non-static method called toString that returns an Object in the form of a String. So if you want that String returned from the Object with getObject () in one line of code, you program this as "String str = getObject (). ToString ();".
Warnings
- Be careful with abstract classes and methods. If a method is "abstract" it cannot be used until it has been executed by another class. This is because an abstract method does not initially contain any code. Abstract classes are used as a kind of framework.