Author:
Christy White
Date Of Creation:
8 May 2021
Update Date:
12 May 2024
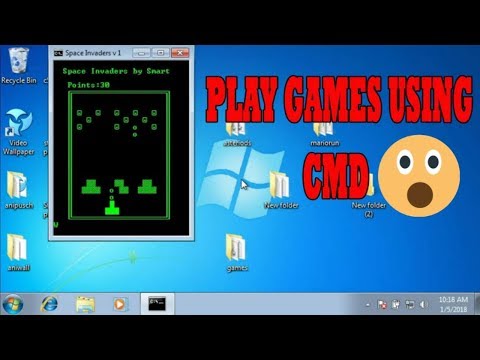
Content
Here's a free and easy way to make a game without having to download anything. You will learn something about batch programming as you go along. You have to make up your own story to give context to this game.
To step
Anything in quotes is subject to change and will not affect the operation of the game - do not change the actual code, however.
Open Notepad or any other text editor - Geany, Notepad ++, etc. Save the file as "My Game ".bat
Start programming. Type the following:
- @echo off
- title "My game"
- color 0A
- if "% 1" neq (goto% 1)
- pause
Add colors to it. Save it and run the code; you see an error message and all the different color combinations. Find the color you like and type it after "color", instead of "zz". A good combination is the color 0A, which makes the text green and the background black.
Create the menu. Create the menu by omitting the pause and typing:
- :Menu
- cls
- echo "1. Start'
- echo "2. Instructions "
- echo "3. Exit "
- set / p answer = "Type the number of your choice and press Enter".
- if% answer% == 1 goto "Start_1"
- if% answer% == 2 goto "Instructions"
- if% answer% == 3 goto "Exit"
Make the "Exit" and "Instructions". Create the Exit screen as follows:
- : "Exit"
- echo Thanks for playing!
- exit / b
- For the instructions, type:
- : "Instructions"
- cls
- echo "Instructions"
- echo.
- And then type:
- echo "Your instructions"
- Enter what you want:
- pause
- goto Menu
Start the game. Type a scenario:
- : Start_1
- cls
- echo "You have run into bandits. Their powers are: "
- echo "3 farmers"
- echo "Your chances of winning are high".
- set / p answer = "Do you want to fight or run?"
- if% answer% == "Fight" goto "Fight_1"
- if% answer% == "Flights" goto "Run_1"
- Fight and flee. Create the fight and flight function as follows:
- : Run_1
- cls
- echo You got out safely!
- pause
- goto "Start_1"
- : Fight_1
- echo You have chosen to fight.
- echo The battle continues.
- set / p answer = Type 1 and press Enter to continue:
- if% answer% == 1 goto Fight_1_Loop
- : "Fight_1_Loop"
- set / a num =% random%
- if% num% gtr 4 goto "Fight_1_Loop"
- if% num% lss 1 goto "Fight_1_Loop"
- if% num% == 1 goto "Lose_Fight_1"
- if% num% == 2 goto "Win_Fight_1"
- if% num% == 3 goto "Win_Fight_1"
- if% num% == 4 goto "Win_Fight_1"
- : "Lose_Fight_1"
- cls
- echo Sorry, you lost the battle :(
- pause
- goto Menu
- : "Win_Fight_1"
- cls
- echo Congratulations, you have won the battle!
- set / p answer = "Do you want to save this?"
- if% answer% == "Yes" goto "Save"
- if% answer% == "No" goto "Start_2"
- : "Save"
- goto "Start_2"
- Now you can repeat the code from "Start_1" to make a second, third or more battles.
- Also keep in mind that if you type Fight_1, for example, you also have to make sure that the parts with the code "goto Fight_1" remain the same, so if you change one, you also have to change the other.
- Close Notepad, click "Yes", save the file, change the file extension in all files and put ".bat" as the extension after the file name.
Tips
- Remember, if you want the player to see something, you have to type "echo" for it.
- Play your game while coding, even if you haven't finished everything yet. This will help you see how what you are typing corresponds to the result and help you identify any errors.
- If you want to stop in the middle of a test of your game, type Ctrl-C.
- Batch files in Windows can be used to automate a variety of tasks, but writing a text game like this is a fun way to learn how it works.
- Check your batch script carefully to make sure you haven't made any mistakes.
- "It often happens that a program will not start.